Recommendation for individuals using a screenreader: please set your punctuation settings to "most."
Descriptive Statements:
- Apply knowledge of characteristics of programming languages (e.g., functional, compiled, object oriented), including block-based languages.
- Apply knowledge of the software development process (e.g., design, testing, maintenance) and methodologies (e.g., waterfall, iterative, agile).
- Apply knowledge of programming style and good programming practices (e.g., indenting, spacing, comments, naming conventions, camelCase) and program documentation.
- Apply knowledge of tools related to the development of computer artifacts (e.g., IDEs, APIs, libraries, mobile device simulators).
- Apply knowledge of common programming errors and procedures for debugging computer programs.
- Demonstrate knowledge of a variety of concepts related to programming (e.g., event driven, heuristic algorithms, parallel processing, artificial intelligence).
Sample Item:
When a smartphone manufacturer releases a new feature, such as fingerprint scanning, the manufacturer needs to provide developers with the ability to incorporate this new feature into their software. This is typically accomplished through the use of:
- Open Systems Interconnection (OSI) model.
- graphical user interface (GUI).
- application programming interface (API).
- Cascading Style Sheets (CSS).
Correct Response and Explanation (Show Correct ResponseHide Correct Response)
C. An API is a set of requirements that determine how one application (app) can talk to another. APIs allow developers limited access to a program's internal functions. In this case, a smartphone manufacturer would typically provide enough access for mobile device app developers to be able to integrate the fingerprint scanner into their apps without exposing all of the fingerprinting application code.
Descriptive Statements:
- Demonstrate knowledge of language-defined data types (e.g., integer, float, Boolean).
- Apply properties of strings and string methods (e.g., length, substring, concatenation).
- Apply knowledge of constants, variables, and classes in various contexts.
- Apply a basic knowledge of data structures (e.g., one-dimensional arrays).
Sample Item:
Use the information below to answer the question that follows.
// string.characterAt(i), returns the character at index i. //
// String index begins at zero. //
email = "last.first@school.edu"
slash slash string.characterAt left parenthesis i right parenthesis, returns the character at index i period slash slash
slash slash String index begins at zero period slash slash
email equals quote last period first at school period edu"
Which of the following methods will return @?
- email.characterAt(8) email period characterAt left parenthesis 8 right parenthesis
- email.characterAt(9) email period characterAt left parenthesis 9 right parenthesis
- email.characterAt(10)email period characterAt left parenthesis 10 right parenthesis
- email.characterAt(11)email period characterAt left parenthesis 11 right parenthesis
Correct Response and Explanation (Show Correct ResponseHide Correct Response)
C. In the string last.first@school.edulast period first at school period edu, the @at is the 11th character in the string. However, since the string index begins at 0, the index of the @at is 10.
Descriptive Statements:
- Apply arithmetical operators as defined by the language (e.g., addition, subtraction, multiplication, integer division, modular arithmetic).
- Apply relational operators in various contexts (e.g., greater than, less than, equal to).
- Interpret logical operators in various contexts (e.g., AND, OR, NOT).
- Apply knowledge of conditional selection structures (e.g., if, if-else), including nesting.
- Apply knowledge of iterative control structures (e.g., while, for), including nesting.
Sample Item:
Use the information below to answer the question that follows.
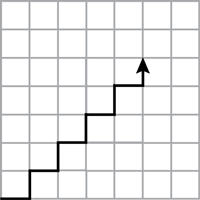
The diagram is a 7 by 7 square grid. A dark line represents the path taken by a robot as seen from above. The line starts in the lower left corner, goes horizontally to the right one square, up one square, to the right one square, up one square, and continues in this pattern to the square in the fifth column over and fifth row to the right. The line ends at this point with an arrow pointing up.
The diagram above shows the path taken by a robot that starts facing in the positive y-direction at the point (0, 0) on a coordinate grid. It can move forward n units (Forward n), turn left 90 degrees (Turn Left), or turn right 90 degrees (Turn Right). Each grid line in the graphic represents 10 units.
Which of the following pseudocode segments will create the path shown in the diagram?
i = 2i equals 2
while (i < 12)while left parenthesis i is less than 12 right parenthesis
if i is even
Turn Right
Forward 10
else
Turn Left
Forward 10
i = i + 1i equals i plus 1
i = 2i equals 2
while (i <= 12)while left parenthesis i is less than or equal to 12 right parenthesis
if i is even
Turn Right
Forward 10
else
Turn Right
Forward 10
i = i + 1i equals i plus 1
i = 2i equals 2
while (i < 12)while left parenthesis i is less than 12 right parenthesis
if i is even
Forward 10
Turn Right
else
Forward 10
Turn Left
i = i + 1i equals i plus 1
i = 2i equals 2
while (i <= 12)while left parenthesis i is less than or equal to 12 right parenthesis
if i is even
Forward 10
Turn Right
else
Forward 10
Turn Left
i = i + 1i equals i plus 1
Correct Response and Explanation (Show Correct ResponseHide Correct Response)
A. In this question, the robot begins at (0, 0), facing in the positive y-direction. To follow the path, the robot must first turn to the right and move 10 units in the positive x-direction, then turn left and move 10 units in the positive y-direction. This is accomplished by turning right when i is even and left when i is odd. Since i begins at 2, and the robot travels for 10 segments, the values for i will be 2 through 11; the loop will not execute once i = 12i equals 12.
Descriptive Statements:
- Demonstrate knowledge of principles of modularization and characteristics of program modules (e.g., functions/methods, objects, classes).
- Analyze the characteristics and uses of inheritance and classes in object- oriented programming.
- Demonstrate knowledge of concepts and principles related to object-oriented design and programming (e.g., abstraction, information hiding, encapsulation, constructors).
- Apply knowledge of function calls, parameters, and parameter-passing techniques.
Sample Item:
Use the pseudocode below to answer the question that follows.
CLASS Flower
private integer numOfPetals
private string color
private bloom()private bloom left parenthesis right parenthesis
public grow()public grow left parenthesis right parenthesis
SUBCLASS Rose inherits from Flower
Rose(numOfPetals, color)Rose left parenthesis numOfPetals, color right parenthesis
MAIN
Rose r1 = Rose(5, "red")Rose r1 equals Rose left parenthesis 5, left quote red right quote right parenthesis
Which of the following demonstrates how to access an element of the class Rose?
- r1.numOfPetalsr1 period numOfPetals
- r1.colorr1 period color
- r1.bloom()r1 period bloomleft parenthesis right parenthesis
- r1.grow()r1 period growleft parenthesis right parenthesis
Correct Response and Explanation (Show Correct ResponseHide Correct Response)
D. In the program, r1 is an object, or instance of class Rose. Rose is a subclass of Flower, and inherits from it. Since Rose is a subclass, it can only access the public variables and methods, in this case the public method, grow()grow left parenthesis right parenthesis. Therefore, r1.grow()r1 period grow left parenthesis right parenthesis is the only way to access an element of r1.